Difference between Onion architecture and clean acrchitecture
September 2, 2023 | by Meir Achildiev
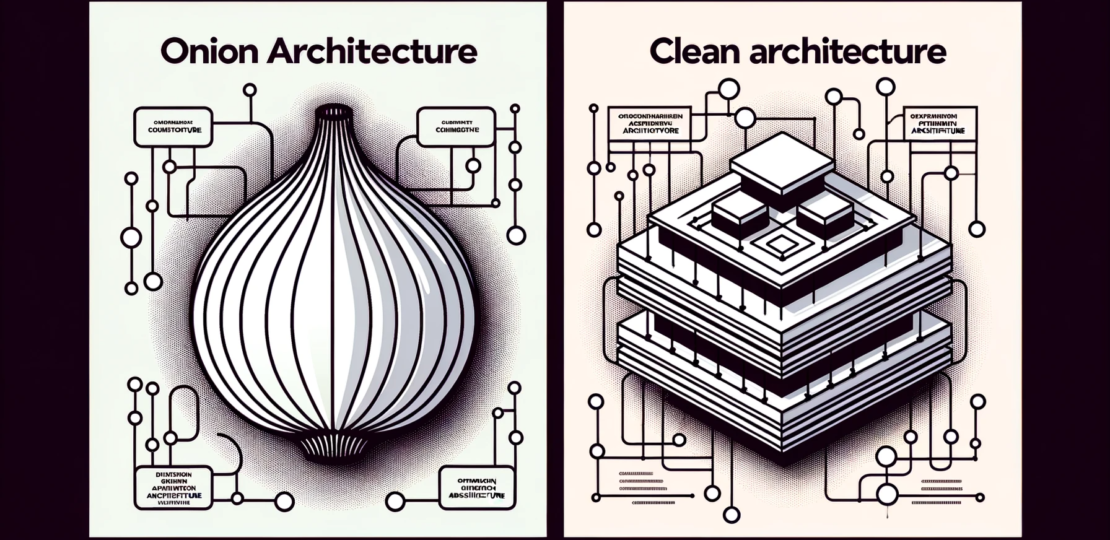
Onion Architecture and Clean Architecture are similar in that they both focus on separation of concerns, maintainability, and testability. Both architectures aim to make the software more understandable, flexible, and maintainable.
Onion Architecture
The layers in Onion Architecture are:
- Domain Layer (Core): This is the innermost layer and includes business logic and types.
- Application Layer: Contains business logic and types.
- Infrastructure Layer: This is where all the I/O components go: UI, Database, Network, etc.
Example:
Domain Layer:
public class User { public Guid Id { get; private set; } public string Name { get; private set; } //other properties and methods }
Infrastructure Layer:
public class UserRepository : IUserRepository { //implementation of the UserRepository }
Pros of Onion Architecture
- Inner layers are independent of outer layers, making the system more flexible and adaptable to changes.
- Testability is high because you can replace outer layers like the infrastructure with test doubles.
- Clear separation of concerns.
- Business logic is at the core and is therefore not affected by changes to the UI or database.
Cons of Onion Architecture
- Might be over-engineered for simple applications.
- Can have a steep learning curve, particularly for developers unfamiliar with it.
- Without careful management, the number of abstraction layers and dependencies can proliferate.
Clean Architecture
Clean Architecture, or Hexagonal Architecture, also has concentric layers but differs slightly:
- Entities: These are the business objects of the application.
- Use Cases: This layer contains specific business rules for the application.
- Controllers: This layer serves as an adapter or converter between the outer layers and the inner layers.
- Devices, Web, UI, External Interfaces: These are all inputs/outputs, or ways the application interacts with the world.
Example:
Entities:
public class User { public Guid Id { get; private set; } public string Name { get; private set; } //other properties and methods }
Use Cases:
public class UserService : IUserService { //implementation of the UserService }
Pros of Clean Architecture
- High degree of testability.
- Business logic and higher-level policies can easily be reused across multiple systems.
- Independence from UI, database, framework, etc.
- Allows changing the database, UI, frameworks without affecting the system.
Cons of Clean Architecture
- Might be overkill for simpler or smaller applications.
- It can be difficult to determine how to correctly implement use cases, entities, etc. for developers unfamiliar with it.
- Requires detailed planning, design, and significant effort upfront.
Similar Architectures
- Hexagonal (Ports and Adapters): Focuses on the inputs and outputs, viewing all mechanisms for inputs and outputs as merely adapters for the core application. This architecture is very similar to Onion and Clean architectures. The main difference is that the Hexagonal Architecture specifically emphasizes the concept of ports (interfaces) and adapters (implementations), while this is a bit more implicit in Onion and Clean architectures.
- Layered (N-tier) Architecture: A traditional architecture that often has UI, Business, and Data layers. It’s simpler but less flexible. Changes can ripple through all the layers, unlike in Onion or Clean where the use of dependency inversion helps prevent this.
In conclusion, there’s a lot of overlap between Onion, Clean, and Hexagonal architectures, and they are all good choices for complex applications requiring maintainability and testability. However, for simpler applications, a more traditional layered architecture may be sufficient. The key is to choose the right tool for the job.
RELATED POSTS
View all