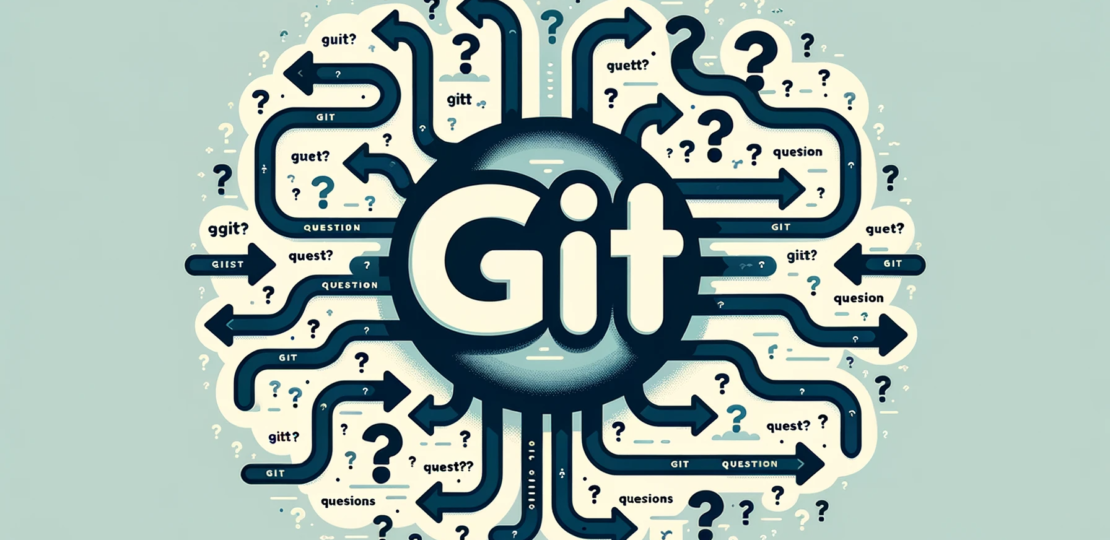
Below are 20 beginner-level questions about Git, complete with answers and examples.
1. What is Git?
Answer: Git is a distributed version control system that helps track changes in source code during software development.
2. How do you initialize a new Git repository?
Answer: You can initialize a new Git repository by running git init
in the root directory of your project.
git init
3. How do you clone a repository?
Answer: Use git clone [URL]
to clone (or copy) a repository from a remote source.
git clone https://github.com/example/repo.git
4. What is a commit?
Answer: A commit is a snapshot of changes in your working directory. Commits provide a history of changes to a project.
5. How do you commit changes?
Answer: Use git commit -m "Your commit message"
to commit changes.
git add . git commit -m "Initial commit"
6. What is a branch?
Answer: A branch is a parallel version of a repository. It’s contained within the repository but does not affect the primary or main
branch.
7. How do you create a new branch?
Answer: Use git branch [branch_name]
to create a new branch.
git branch new-feature
8. How do you switch between branches?
Answer: Use git checkout [branch_name]
to switch branches.
git checkout new-feature
9. How do you merge branches?
Answer: Use git merge [branch_name]
to merge the specified branch into the current branch.
git merge new-feature
10. How do you update your local repository?
Answer: Use git pull
to update the local repository with changes from the remote repository.
git pull origin main
11. What is HEAD
in Git?
Answer: HEAD
is a reference to the last commit in the currently checked-out branch.
12. What is a remote in Git?
Answer: A remote is a Git repository hosted on the internet or another network.
13. How do you add a remote?
Answer: Use git remote add [name] [url]
to add a new remote.
git remote add origin https://github.com/example/repo.git
14. How do you view all branches?
Answer: Use git branch -a
to list all branches, including remote ones.
git branch -a
15. What is a conflict in Git?
Answer: A conflict occurs when different branches have changes to the same line in a file, or when a file is deleted in one branch but edited in another.
16. How do you resolve a conflict?
Answer: Conflicts can be resolved manually by editing the conflicted file, or automatically with tools.
17. What is git stash
?
Answer: git stash
temporarily saves changes that have not been committed so that you can switch branches.
git stash
18. What is git revert
?
Answer: git revert
creates a new commit that undoes changes made in a specified commit.
git revert [commit_hash]
19. What does git push
do?
Answer: git push
uploads local repository changes to a remote repository.
git push origin main
20. What is git fetch
?
Answer: git fetch
downloads objects and refs from another repository but does not integrate any of this new data onto your working files.
git fetch origin
21. What is .gitignore
?
Answer: A .gitignore
file specifies files and directories that should be ignored by Git and not included in the version control system.
Example:
# .gitignore *.log node_modules/
22. How do you remove a file from Git?
Answer: Use git rm [file_name]
to remove a file from the Git repository and stage the removal for commit.
Example:
git rm unwanted-file.txt
23. How do you rename a file in Git?
Answer: Use git mv [old_name] [new_name]
to rename a file.
Example:
git mv oldname.txt newname.txt
24. What is a ‘Fork’ in Git?
Answer: A fork is a copy of a repository that belongs to you, allowing you to make changes without affecting the original project.
25. How do you list all the remote repositories?
Answer: Use git remote -v
to list all the remote repositories.
Example:
git remote -v
26. What is git log
?
Answer: git log
shows the commit history in the current repository.
Example:
git log
27. How do you undo the last commit?
Answer: You can use git reset HEAD~1
to undo the last commit.
Example:
git reset HEAD~1
28. What is a ‘Tag’ in Git?
Answer: A tag in Git is a way to mark a specific commit, usually to denote a release or milestone.
Example:
git tag v1.0.0
29. How do you list all tags?
Answer: Use git tag
to list all tags.
Example:
git tag
30. What is git diff
?
Answer: git diff
shows differences between commits, branches, or your working directory and a branch.
Example:
git diff
31. What is git blame
?
Answer: git blame
shows who made each change in a file and when.
Example:
git blame myfile.txt
32. How do you add all changes in a directory?
Answer: Use git add .
to stage all new and modified files for commit.
Example:
git add .
33. What is git cherry-pick
?
Answer: git cherry-pick
applies the changes from an existing commit to the current branch.
Example:
git cherry-pick [commit_hash]
34. What is a submodule?
Answer: A submodule allows you to keep another Git repository in a subdirectory of your repository.
35. How do you initialize and update a submodule?
Answer: Use git submodule init
and git submodule update
to initialize and update submodules.
git submodule init git submodule update
36. What is git rebase
?
Answer: git rebase
is used to move a series of commits to a new base commit, effectively reapplying changes on top of another branch.
Example:
git rebase main
37. What does git status
do?
Answer: git status
shows the status of changes in the working directory.
Example:
git status
38. How do you set your Git username and email?
Answer: Use git config
to set your Git username and email.
Example:
git config --global user.name "Your Name" git config --global user.email "youremail@example.com"
39. What is git stash apply
?
Answer: git stash apply
applies changes stored in a stash to your working directory without deleting the stash.
Example:
git stash apply
40. What is git show
?
Answer: git show
displays information about a Git object, like a commit or a tag.
Example:
git show [commit_hash]
RELATED POSTS
View all