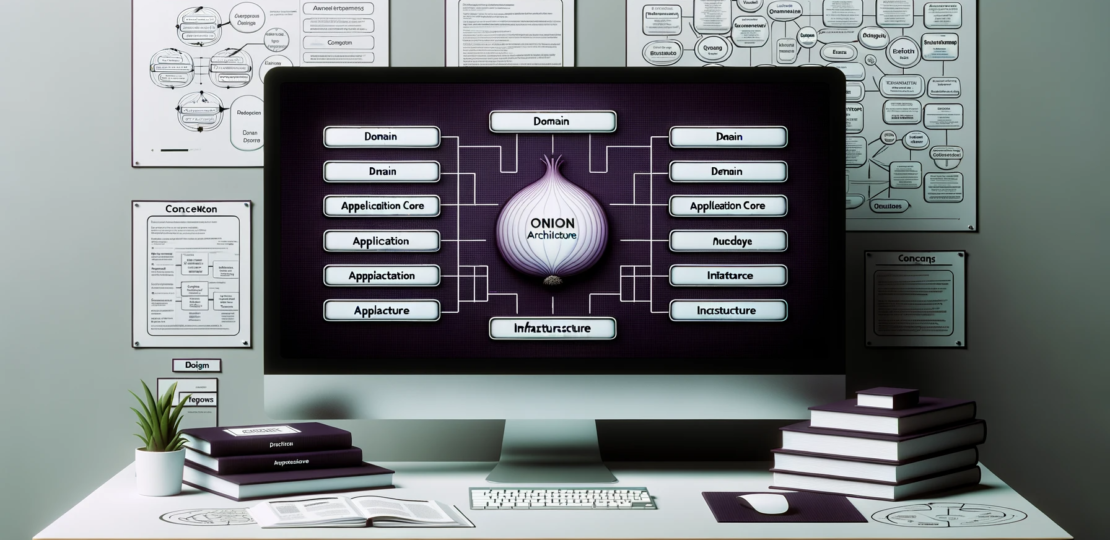
Conceptual Understanding
- Onion Architecture is about the positioning of concerns and flow of dependencies, primarily toward the center. Dependencies flow inward, not outward.
- The center of the onion, the Domain layer, should have no dependencies on any outer layers. It defines the business rules and data.
- The layers include: Domain Model (center), Application Layer, Infrastructure (outermost).
- The inner layers define interfaces that outer layers implement, leading to an architecture that is easy to maintain, easy to test, and less coupled.
- The infrastructure is just a detail and the application should not be dependent on it.
Domain Layer
- This is the innermost layer representing enterprise-wide business concepts.
- It includes Entities, Value Objects, and Domain Events.
Example 1:
//Entity public class User { public Guid Id { get; private set; } public string Email { get; private set; } public string Name { get; private set; } private User() { } public User(string email, string name) { //business rules go here if (string.IsNullOrEmpty(email) || string.IsNullOrEmpty(name)) throw new ArgumentException("Email and Name cannot be null or empty."); Id = Guid.NewGuid(); Email = email; Name = name; } }
- It is technology-agnostic and has no dependencies on outer layers.
- Contains business rules that should be common to multiple applications in the same domain.
- Defines interfaces for repositories that outer layers will implement.
Example 2:
//Interface for repository public interface IUserRepository { Task AddAsync(User user); Task<User> GetByEmailAsync(string email); }
Application Layer
- Contains use case specific business rules.
- This layer orchestrates the domain layer and the infrastructure layer.
- It’s responsible for calling infrastructure layer interfaces like repository interfaces or infrastructure services.
- It communicates with the domain layer using interfaces.
- Services in this layer serve as the entry point to the application.
Example 3:
public class UserService { private readonly IUserRepository _userRepository; public UserService(IUserRepository userRepository) { _userRepository = userRepository; } public async Task CreateUserAsync(string email, string name) { var existingUser = await _userRepository.GetByEmailAsync(email); if (existingUser != null) throw new ArgumentException("User with the same email already exists."); var user = new User(email, name); await _userRepository.AddAsync(user); } }
Infrastructure Layer
- This is the outermost layer.
- It provides concrete implementations for interfaces defined in the inner layers, like database repositories, file system interactions, network communication, etc.
- It communicates with the inner layers through interfaces.
- This layer should be easily replaceable.
- It may contain implementations of domain services if they involve infrastructure concerns.
Example 4:
public class UserRepository : IUserRepository { private readonly MyDbContext _context; public UserRepository(MyDbContext context) { _context = context; } public async Task AddAsync(User user) { await _context.Users.AddAsync(user); await _context.SaveChangesAsync(); } public async Task<User> GetByEmailAsync(string email) { return await _context.Users.FirstOrDefaultAsync(u => u.Email == email); } }
In this example, MyDbContext
would be your Entity Framework Core DbContext, which is a part of the Infrastructure layer and provides a gateway to your database.
Architectural Advantages
- High level of maintainability due to the decoupled nature of the architecture.
- Highly testable as dependencies can be easily mocked.
- Independent of technology, making it flexible and adaptable to changes.
- Changes in the database or UI do not affect the core logic of the application.
Architectural Disadvantages
- It can be an overkill for small applications, adding unnecessary complexity.
- Can be time-consuming to set up due to the strict separation of concerns.
The Onion Architecture promotes separation of concerns, maintainability, and testability, making it a great choice for large and complex applications. However, always analyze your project’s requirements to ensure that this architectural style is a suitable choice.
RELATED POSTS
View all