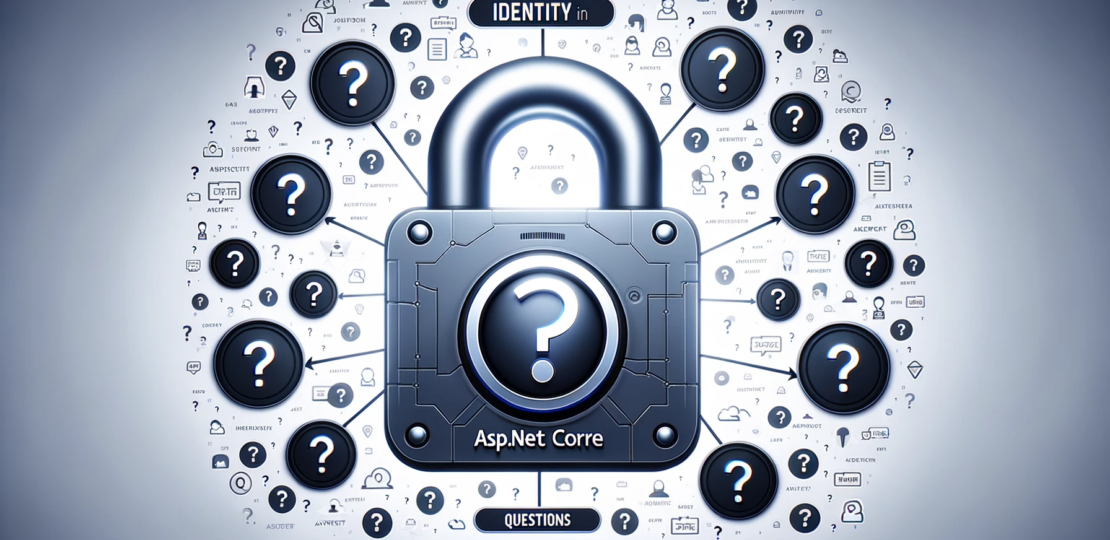
40 questions and answers regarding Identity in ASP.NET Core:
- What is ASP.NET Core Identity?
ASP.NET Core Identity is a membership system which allows you to add login functionality to your application. Users can create an account and sign in through a variety of providers. ASP.NET Core Identity provides features for password hashing, password policy enforcement, OAuth 2.0, Two-Factor Authentication (2FA), account confirmation, claims management, and more. - How do you create an ASP.NET Core application with Identity?
You can use the .NET CLI or Visual Studio to create a new ASP.NET Core project with Identity:
dotnet new webapp --auth Individual
This command creates an ASP.NET Core Razor Pages application with Individual User Accounts.
- How do you add Identity to an existing project?
First, you need to install the Identity package:
dotnet add package Microsoft.AspNetCore.Identity.EntityFrameworkCore
Then, create a new User class:
public class ApplicationUser : IdentityUser { }
And then create a new context class:
public class ApplicationDbContext : IdentityDbContext<ApplicationUser> { public ApplicationDbContext(DbContextOptions<ApplicationDbContext> options) : base(options) { } }
Finally, register the context and the Identity services in Startup.cs
:
services.AddDbContext<ApplicationDbContext>(options => options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection"))); services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>();
- How do you handle password complexity rules in Identity?
You can configure the password complexity rules in theStartup.cs
file:
services.Configure<IdentityOptions>(options => { // Password settings. options.Password.RequireDigit = true; options.Password.RequireLowercase = true; options.Password.RequireNonAlphanumeric = true; options.Password.RequireUppercase = true; options.Password.RequiredLength = 6; options.Password.RequiredUniqueChars = 1; });
- How do you enable Two-Factor Authentication in Identity?
You can enable Two-Factor Authentication (2FA) in Identity by setting theTwoFactorEnabled
property totrue
:
var user = await _userManager.GetUserAsync(User); await _userManager.SetTwoFactorEnabledAsync(user, true);
Then, you need to verify the second factor (e.g., a verification code sent via SMS or email).
- How do you customize the Identity user model?
You can extend theIdentityUser
class to include additional properties:
public class ApplicationUser : IdentityUser { public string FullName { get; set; } }
Then use your ApplicationUser
class instead of IdentityUser
.
- How do you add custom claims to the Identity user?
You can add custom claims to a user with theUserManager
class:
var claim = new Claim("DateOfBirth", "1970-01-01"); var result = await _userManager.AddClaimAsync(user, claim);
- How do you check if a user is in a role?
You can use theUserManager
class to check if a user is in a role:
var isInRole = await _userManager.IsInRoleAsync(user, "Admin");
- How do you create roles in Identity?
You can use theRoleManager
class to create roles:
var role = new IdentityRole("Admin"); var result = await _roleManager.CreateAsync(role);
- How do you add a user to a role?
You can use theUserManager
class to add a user to a role:
var result = await _userManager.AddToRoleAsync(user, "Admin");
- How do you remove a user from a role?
You can use theUserManager
class to remove a user from a role:
var result = await _userManager.RemoveFromRoleAsync(user, "Admin");
- How do you use the [Authorize] attribute with roles?
You can restrict access to a controller or action to users with a specific role:
[Authorize(Roles = "Admin")] public class AdminController : Controller { // ... }
- How do you register a user in Identity?
You can use theUserManager
class to register a user:
var user = new ApplicationUser { UserName = model.Email, Email = model.Email }; var result = await _userManager.CreateAsync(user, model.Password);
- How do you sign in a user in Identity?
You can use theSignInManager
class to sign in a user:
var result = await _signInManager.PasswordSignInAsync(model.Email, model.Password, model.RememberMe, lockoutOnFailure: false);
- How do you sign out a user in Identity?
You can use theSignInManager
class to sign out a user:
await _signInManager.SignOutAsync();
- How do you enable email confirmation in Identity?
First, generate a confirmation token for a user:
var code = await _userManager.GenerateEmailConfirmationTokenAsync(user);
Then, send the token to the user via email. The user clicks the link and is redirected to your app, where you confirm their email:
var result = await _userManager.ConfirmEmailAsync(user, code);
- How do you enable password reset in Identity?
First, generate a password reset token for a user:
var code = await _userManager.GeneratePasswordResetTokenAsync(user);
Then, send the token to the user via email. The user clicks the link and is redirected to your app, where they can reset their password:
var result = await _userManager.ResetPasswordAsync(user, code, model.Password);
- How do you change a user’s password in Identity?
You can use theUserManager
class to change a user’s password:
var result = await _userManager.ChangePasswordAsync(user, model.OldPassword, model.NewPassword);
- How do you customize the Identity UI?
You can scaffold the Identity views and modify them:
dotnet aspnet-codegenerator identity --useDefaultUI
Then, you can modify the generated Razor views in the Areas/Identity/Pages
directory.
- How do you lock out a user in Identity?
You can use theUserManager
class to lock out a user:
var result = await _userManager.SetLockoutEndDateAsync(user, DateTimeOffset.UtcNow.AddMinutes(15));
- How do you check if a user is locked out in Identity?
You can use theUserManager
class to check if a user is locked out:
var isLockedOut = await _userManager.IsLockedOutAsync(user);
- How do you disable user lockout in Identity?
You can configure the lockout settings inStartup.cs
:
services.Configure<IdentityOptions>(options => { options.Lockout.AllowedForNewUsers = false; });
- How do you use policy-based authorization in Identity?
First, define a policy inStartup.cs
:
services.AddAuthorization(options => { options.AddPolicy("AtLeast18", policy => policy.Requirements.Add(new MinimumAgeRequirement(18))); });
Then, create a AuthorizationHandler
for the MinimumAgeRequirement
:
public class MinimumAgeHandler : AuthorizationHandler<MinimumAgeRequirement> { protected override Task HandleRequirementAsync(AuthorizationHandlerContext context, MinimumAgeRequirement requirement) { if (context.User.HasClaim(c => c.Type == ClaimTypes.DateOfBirth)) { var dateOfBirth = Convert.ToDateTime(context.User.FindFirst(c => c.Type == ClaimTypes.DateOfBirth).Value); var age = DateTime.Today.Year - dateOfBirth.Year; if (age >= requirement.MinimumAge) { context.Succeed(requirement); } } return Task.CompletedTask; } }
Finally, apply the policy to a controller or action:
[Authorize(Policy = "AtLeast18")] public class AccountController : Controller { // ... }
- How do you use external authentication providers in Identity?
You can configure external authentication providers (such as Google, Facebook, Twitter, etc.) inStartup.cs
:
services.AddAuthentication() .AddGoogle(options => { options.ClientId = Configuration["Authentication:Google:ClientId"]; options.ClientSecret = Configuration["Authentication:Google:ClientSecret"]; });
Then, you can add a button to your login page to sign in with Google:
<a class="btn btn-primary" href="/Identity/Account/ExternalLogin?provider=Google&returnUrl=%2F">Sign in with Google</a>
- How do you handle email verification for external logins in Identity?
After a user signs in with an external provider, you can ask them to confirm their email:
var info = await _signInManager.GetExternalLoginInfoAsync(); var email = info.Principal.FindFirstValue(ClaimTypes.Email); var user = new ApplicationUser { UserName = email, Email = email }; var result = await _userManager.CreateAsync(user); if (result.Succeeded) { var code = await _userManager.GenerateEmailConfirmationTokenAsync(user); // Send the email confirmation code }
- How do you customize the Identity cookie?
You can customize the Identity cookie inStartup.cs
:
services.ConfigureApplicationCookie(options => { options.Cookie.HttpOnly = true; options.ExpireTimeSpan = TimeSpan.FromMinutes(60); options.LoginPath = "/Identity/Account/Login"; options.LogoutPath = "/Identity/Account/Logout"; });
- How do you add custom validators in Identity?
You can create a class that implementsIUserValidator<ApplicationUser>
orIPasswordValidator<ApplicationUser>
:
public class CustomUserValidator : IUserValidator<ApplicationUser> { public Task<IdentityResult> ValidateAsync(UserManager<ApplicationUser> manager, ApplicationUser user) { if (user.Email.ToLower().EndsWith("@example.com")) { return Task.FromResult(IdentityResult.Failed(new IdentityError { Code = "EmailDomainError", Description = "Only example.com email addresses are allowed", })); } else { return Task.FromResult(IdentityResult.Success); } } }
Then, register your custom validator in Startup.cs
:
services.AddTransient<IUserValidator<ApplicationUser>, CustomUserValidator>();
- How do you handle account lockout in Identity?
You can configure the account lockout settings inStartup.cs
:
services.Configure<IdentityOptions>(options => { options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(5); options.Lockout.MaxFailedAccessAttempts = 5; options.Lockout.AllowedForNewUsers = true; });
Then, handle account lockout in your login action:
var result = await _signInManager.PasswordSignInAsync(model.Email, model.Password, model.RememberMe, lockoutOnFailure: true); if (result.IsLockedOut) { _logger.LogWarning("User account locked out."); return RedirectToPage("./Lockout"); }
- How do you set up password recovery in Identity?
First, generate a password reset token for a user:
var code = await _userManager.GeneratePasswordResetTokenAsync(user);
Then, send the token to the user via email. The user clicks the link and is redirected to your app, where they can reset their password:
var result = await _userManager.ResetPasswordAsync(user, code, model.Password);
- How do you enable account confirmation in Identity?
First, generate an email confirmation token for a user:
var code = await _userManager.GenerateEmailConfirmationTokenAsync(user);
Then, send the token to the user via email. The user clicks the link and is redirected to your app, where you confirm their email:
var result = await _userManager.ConfirmEmailAsync(user, code);
- How do you handle username uniqueness in Identity?
By default, Identity ensures that usernames are unique. When you try to create a user with a username that already exists,UserManager.CreateAsync
returns anIdentityResult
with aSucceeded
property offalse
:
var user = new ApplicationUser { UserName = model.Email, Email = model.Email }; var result = await _userManager.CreateAsync(user, model.Password); if (!result.Succeeded) { foreach (var error in result.Errors) { ModelState.AddModelError(string.Empty, error.Description); } }
- How do you handle email uniqueness in Identity?
You can configure Identity to require unique emails inStartup.cs
:
services.Configure<IdentityOptions>(options => { options.User.RequireUniqueEmail = true; });
- How do you use JWT authentication with Identity? You can configure JWT authentication in
Startup.cs
:
services.AddAuthentication(options => { options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme; options.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme; }) .AddJwtBearer(options => { options.TokenValidationParameters = new TokenValidationParameters { ValidateIssuerSigningKey = true, IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["Jwt:Key"])), ValidateIssuer = false, ValidateAudience = false, }; });
Then, generate a JWT when a user logs in:
var claims = new[] { new Claim(JwtRegisteredClaimNames.Sub, user.UserName), new Claim(JwtRegisteredClaimNames.Jti, Guid.NewGuid().ToString()), }; var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["Jwt:Key"])); var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256); var token = new JwtSecurityToken( issuer: Configuration["Jwt:Issuer"], audience: Configuration["Jwt:Issuer"], claims: claims, expires: DateTime.Now.AddMinutes(30), signingCredentials: creds); return new JwtSecurityTokenHandler().WriteToken(token);
- How do you sign out a user in Identity?
You can sign out a user usingSignInManager
:
await _signInManager.SignOutAsync();
- How do you handle two-factor authentication in Identity?
First, enable two-factor authentication for a user:
var result = await _userManager.SetTwoFactorEnabledAsync(user, true);
Then, when a user logs in, send them a two-factor code:
var code = await _userManager.GenerateTwoFactorTokenAsync(user, provider);
Finally, verify the code when the user submits it:
var result = await _signInManager.TwoFactorSignInAsync(provider, model.Code, isPersistent: false, rememberClient: false);
- How do you add custom user claims in Identity?
You can add custom user claims usingUserManager
:
var claim = new Claim("Department", "Engineering"); var result = await _userManager.AddClaimAsync(user, claim);
- How do you check a user’s role in Identity?
You can check a user’s role usingUserManager
:
var isInRole = await _userManager.IsInRoleAsync(user, "Admin");
- How do you check a user’s claim in Identity?
You can check a user’s claim by examining theClaimsIdentity
:
var identity = (ClaimsIdentity)User.Identity; var hasClaim = identity.HasClaim(c => c.Type == "Department" && c.Value == "Engineering");
- How do you delete a user in Identity?
You can delete a user usingUserManager
:
var result = await _userManager.DeleteAsync(user);
- How do you update a user’s password in Identity?
You can update a user’s password usingUserManager
:
var result = await _userManager.ChangePasswordAsync(user, model.CurrentPassword, model.NewPassword);
RELATED POSTS
View all