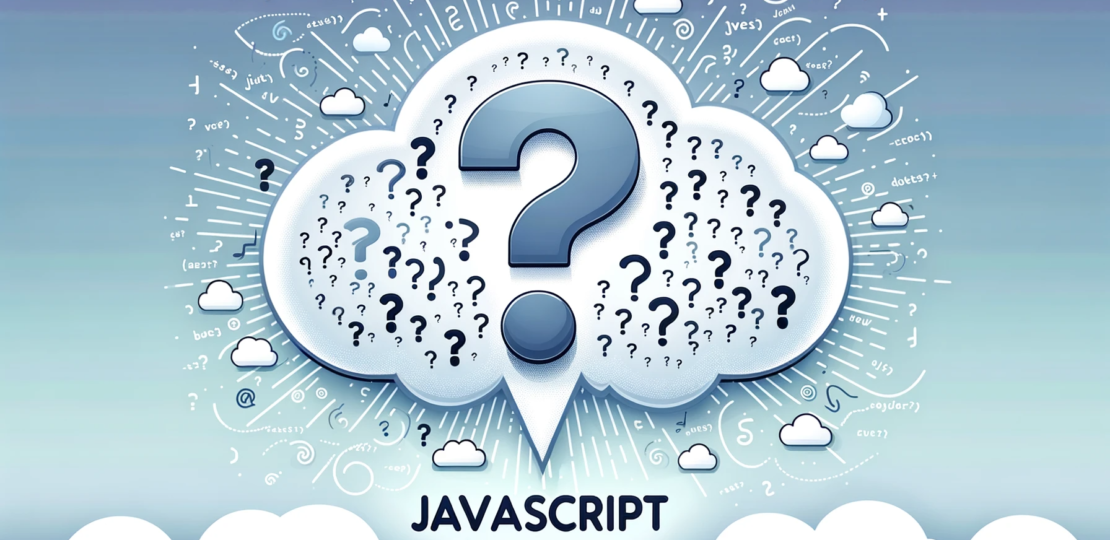
Here are 50 simple Javascript questions along with their answers and code examples:
- What is the output of
console.log(5 + "5")
in JavaScript?- This will output
55
. Because one operand is a string, JavaScript will convert the other operand to a string before performing the concatenation.
- This will output
- What is the output of
console.log(5 - "5")
in JavaScript?- This will output
0
. JavaScript performs type coercion and converts the string"5"
to the number5
, then does the subtraction.
- This will output
- What is the output of
console.log(5 == "5")
in JavaScript?- This will output
true
. The double equals==
operator performs type coercion if the types on both sides are different.
- This will output
- What is the output of
console.log(5 === "5")
in JavaScript?- This will output
false
. The triple equals===
operator does not perform type coercion.
- This will output
- Write a function to reverse a string in JavaScript.
function reverseString(str) { return str.split('').reverse().join(''); }
- How do you declare a variable in JavaScript?
var myVariable = "Hello"; let myVariable = "Hello"; const myVariable = "Hello";
- What is a callback function in JavaScript? A callback function is a function passed into another function as an argument, which is then invoked inside the outer function.
function greet(name, callback) { console.log('Hello ' + name); callback(); } greet('John', function() { console.log('This is a callback'); });
- What is closure in JavaScript?
- A closure is a function having access to the parent scope, even after the parent function has closed.
function outer() { var x = 10; function inner() { console.log(x); } return inner; } var innerFunc = outer(); innerFunc(); // Output: 10
- How do you write a for loop in JavaScript?
for (var i = 0; i < 5; i++) { console.log(i); }
- How do you write an if-else statement in JavaScript?
var x = 10; if (x > 5) { console.log("x is greater than 5"); } else { console.log("x is not greater than 5"); }
- How do you declare a function in JavaScript?
function myFunction() { console.log("Hello World"); }
- How do you add an item to an array in JavaScript?
var arr = [1, 2, 3]; arr.push(4);
- How do you remove an item from an array in JavaScript?
var arr = [1, 2, 3]; arr.pop(); // Removes last element
- How do you check if a variable is an array in JavaScript?
var arr = [1, 2, 3]; console.log(Array.isArray(arr)); // true
- How can you concatenate strings in JavaScript?
var str1 = "Hello"; var str2 = "World"; console.log(str1 + " " + str2); // "Hello World"
- How do you declare an object in JavaScript?
var obj = { name: "John", age: 30 };
- How do you access properties of an object in JavaScript?
var obj = { name: "John", age: 30 }; console.log(obj.name); // "John" console.log(obj['age']); // 30
- What is the output of
console.log(null == undefined)
in JavaScript?- This will output
true
because==
operator performs type coercion and bothnull
andundefined
are falsy values.
- This will output
- What is the output of
console.log(null === undefined)
in JavaScript?- This will output
false
because===
operator doesn’t perform type coercion.
- This will output
- How do you check if a variable is undefined in JavaScript?
var myVar; if (typeof myVar === 'undefined') { console.log('myVar is undefined'); }
- What is ‘use strict’ in JavaScript?
'use strict'
is a directive introduced in ECMAScript 5. It helps catch common coding mistakes and “unsafe” actions by throwing exceptions.
'use strict'; x = 3.14; // This will throw an error because x is not declared
- What is the difference between
let
,const
, andvar
?var
is function scoped, and if it is declared outside a function it is globally scoped.let
andconst
are block scoped.const
is a read-only reference to a value.
- What are arrow functions in JavaScript?
- Arrow functions are a shorthand syntax for writing function expressions. They cannot be used as constructors and do not have their own
this
,arguments
,super
, ornew.target
.
- Arrow functions are a shorthand syntax for writing function expressions. They cannot be used as constructors and do not have their own
const sum = (a, b) => a + b;
- What are JavaScript Promises?
- A Promise in JavaScript is an object representing the eventual completion or failure of an asynchronous operation. A Promise is in one of three states: pending, fulfilled, or rejected.
let promise = new Promise(function(resolve, reject) { // the function is executed automatically when the promise is constructed // after 1 second signal that the job is done with the result "done" setTimeout(() => resolve("done"), 1000); });
- What is a JavaScript Promise
then
method?- The
then
method returns a Promise. It takes two arguments, both are callback functions for the success and failure cases respectively.
- The
const promise = new Promise((resolve, reject) => { setTimeout(() => { resolve("Resolved!"); }, 1000); }); promise.then(value => console.log(value)); // "Resolved!"
- What is a JavaScript Promise
catch
method?- The
catch
method returns a Promise and deals with rejected cases only.
- The
const promise = new Promise((resolve, reject) => { setTimeout(() => { reject("Rejected!"); }, 1000); }); promise.catch(reason => console.log(reason)); // "Rejected!"
- What is JavaScript `async/await`?
The async
function declaration defines an asynchronous function, which returns an AsyncFunction object. The await
operator is used to wait for a Promise.
async function myFunction() { const promise = new Promise((resolve, reject) => { setTimeout(() => resolve("Hello"), 1000); }); const result = await promise; // wait until the promise resolves console.log(result); // "Hello" } myFunction();
- What is the difference between
==
and===
in JavaScript?- The
==
operator will compare for equality after doing any necessary type conversions. The===
operator will not do the conversion, so if two values are not the same type===
will simply returnfalse
.
- The
- How do you add a property to an object in JavaScript?
var obj = { name: "John", age: 30 }; obj.job = "Developer"; console.log(obj); // {name: "John", age: 30, job: "Developer"}
- How do you delete a property from an object in JavaScript?
var obj = { name: "John", age: 30, job: "Developer" }; delete obj.job; console.log(obj); // {name: "John", age: 30}
- What is
NaN
in JavaScript?NaN
is a special value in JavaScript that stands for “Not a Number”. It is the returned value when Math functions fail or when a function trying to parse a number fails.
console.log(0 / 0); // NaN
- How do you check if a value is
NaN
in JavaScript?
console.log(isNaN(0 / 0)); // true
- What is an IIFE (Immediately Invoked Function Expression) in JavaScript?
- An IIFE is a JavaScript function that runs as soon as it is defined.
(function() { console.log('Hello World'); })();
- What is the difference between null and undefined in JavaScript?
null
is an assignment value. It means no value or no object. It is not an identifier for a property of an object or for a variable itself.undefined
means a variable has been declared but has not yet been assigned a value.
- How to get a random number between 1 and 100 in JavaScript?
var randomNum = Math.floor(Math.random() * 100) + 1; console.log(randomNum);
- How can you convert a string to an integer in JavaScript?
var str = "123"; var int = parseInt(str); console.log(int); // 123
- How can you convert a string to a number in JavaScript?
var str = "123.45"; var num = parseFloat(str); console.log(num); // 123.45
- What are JavaScript data types?
- JavaScript data types include Number, String, Boolean, Object, Function, Null, Undefined, and Symbol (in ES6).
- How can you get the type of a variable in JavaScript?
var str = "Hello"; console.log(typeof str); // "string"
- What is JSON in JavaScript?
- JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
- How do you parse JSON in JavaScript?
var json = '{"name":"John", "age":30, "city":"New York"}'; var obj = JSON.parse(json); console.log(obj.name); // "John"
- How do you convert an object to a JSON string in JavaScript?
var obj = {name: "John", age: 30, city: "New York"}; var json = JSON.stringify(obj); console.log(json); // '{"name":"John", "age":30, "city":"New York"}'
- How do you create a date object in JavaScript?
var date = new Date(); console.log(date);
- How do you get the current time in JavaScript?
var date = new Date(); console.log(date.getTime());
- How do you get the current date in JavaScript?
var date = new Date(); console.log(date.toDateString());
- What are JavaScript events?
- JavaScript events are actions that can be detected by JavaScript. For example, a user clicking a button is an event.
- How do you prevent the default action of an event in JavaScript?
element.addEventListener('click', function(event) { event.preventDefault(); // do something });
- How do you attach an event handler to an element in JavaScript?
var button = document.querySelector('button'); button.addEventListener('click', function() { console.log('Button clicked'); });
- How do you get the value of an input field in JavaScript?
var input = document.querySelector('input'); console.log(input.value);
- How do you change the content of an HTML element in JavaScript?
var element = document.querySelector('p'); element.textContent = 'New content';
I hope these questions will help you in learning JavaScript. Happy coding!
RELATED POSTS
View all